Welcome to our comprehensive ESP32 pinout guide! In this article, we will walk you through the intricacies of the ESP32 pinout and help you understand the functions of each pin. By familiarizing yourself with the pinout, you will be able to make the most of your ESP32 projects and unleash their full potential.
ESP32 Pinout Overview
In this section, we will provide an overview of the ESP32 pinout, discussing the various pins available on the ESP32 and their general purposes. Understanding the layout and arrangement of the pins will be crucial for working with the ESP32 effectively.
The ESP32 is a powerful microcontroller with a wide range of capabilities, and its pinout consists of various types of pins that serve different functions. By understanding the pinout, you will be able to leverage the full potential of the ESP32 for your projects.
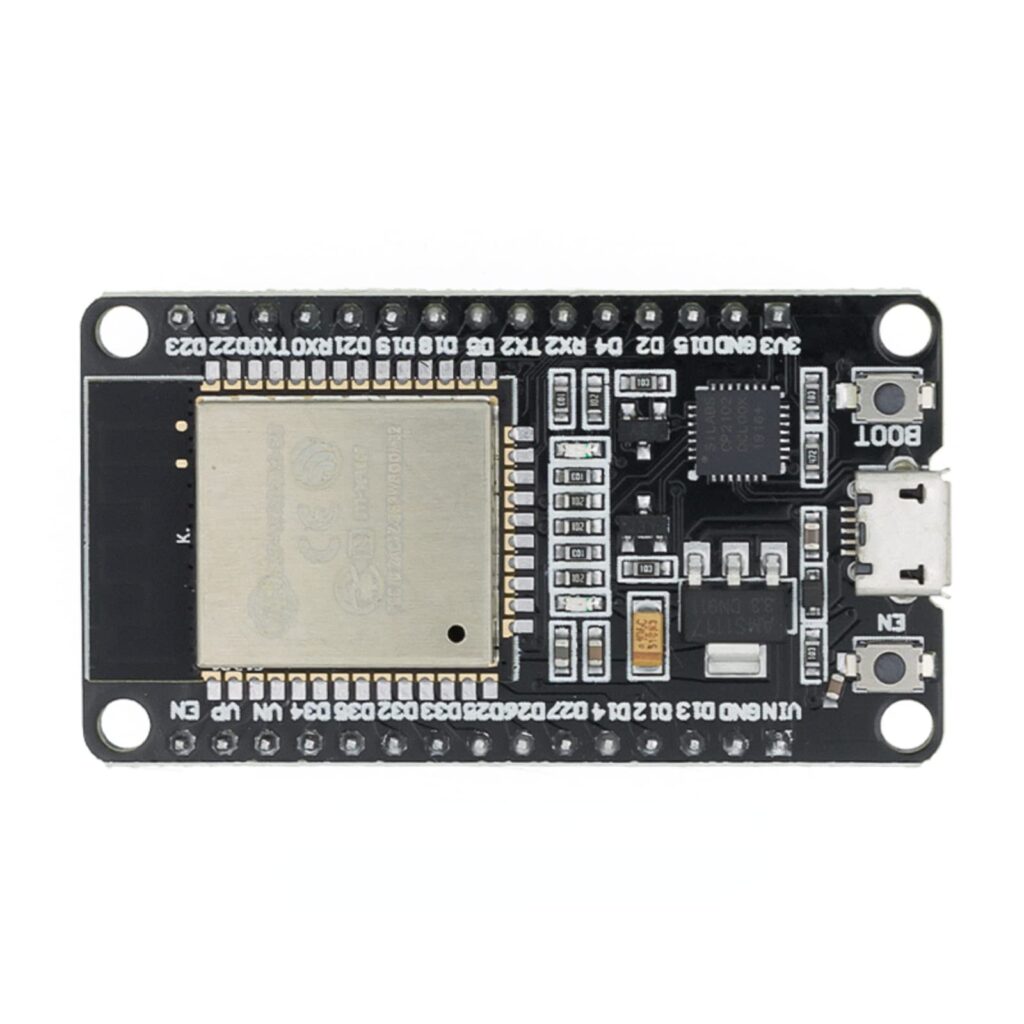
Diving into the Pinout
The ESP32 pinout is designed to provide flexibility and versatility, allowing you to connect and control a wide variety of components and peripherals. It features a combination of digital, analog, communication, and power pins that cater to different project requirements.
Here are the main types of pins you will find in the ESP32 pinout:
- Digital Pins: These pins can be configured as inputs or outputs, allowing you to read digital signals from sensors or control digital devices.
- Analog Pins: These pins enable analog input and output, allowing you to read and generate analog values for precise measurements or control.
- Communication Pins: These pins support various communication protocols such as UART, SPI, and I2C, enabling you to connect and communicate with other devices or modules.
- Power Pins: These pins provide power supply options for the ESP32 and allow you to connect external power sources to the board.
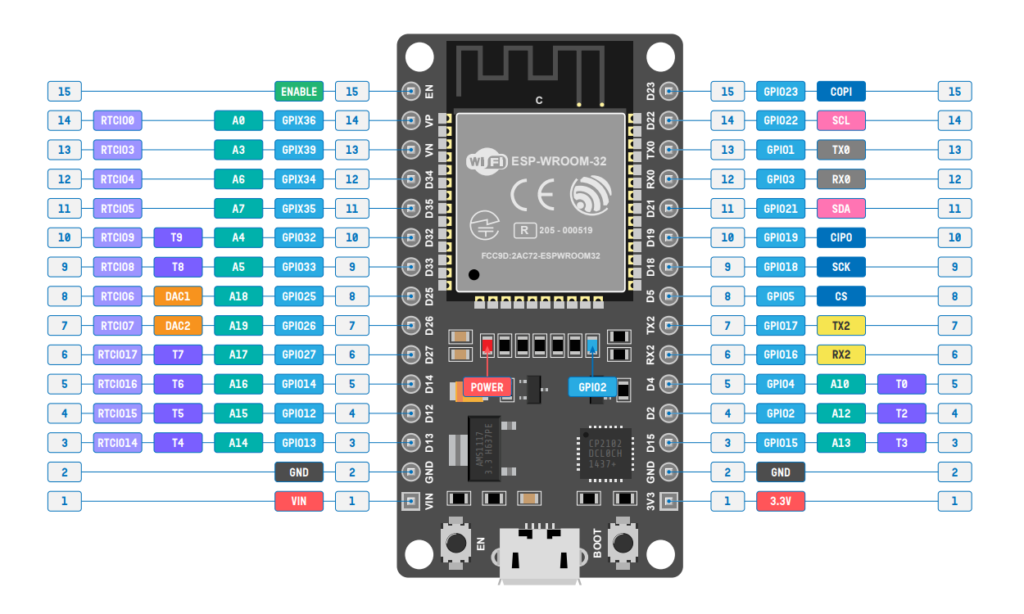
Digital Pins
In this section, we will delve into the digital pins of the ESP32 board. Digital pins are versatile and essential for interfacing with other devices and components. They can be used for both input and output functions, making them incredibly useful for a wide range of projects.
One of the primary functions of digital pins is to read and write binary values, representing logic high (1) or logic low (0). This allows you to communicate with other digital devices and control their operation.
For example, let’s say you want to control an LED using the ESP32. You can connect the LED to one of the digital pins and use code to turn it on or off:
#define LED_PIN 12
pinMode(LED_PIN, OUTPUT);
digitalWrite(LED_PIN, HIGH); // Turns on the LED
delay(1000);
digitalWrite(LED_PIN, LOW); // Turns off the LED
As seen in the example, the pinMode function is used to configure the digital pin as an output, while digitalWrite is used to set the pin’s state (HIGH or LOW).
Furthermore, digital pins can also be configured as inputs to read signals from external devices or sensors. By using functions like pinMode and digitalRead, you can detect the state of a button, read data from a sensor, or listen for a signal from another device.
For instance, suppose you have a push-button connected to a digital pin. You can use the following code to detect when the button is pressed:
#define BUTTON_PIN 5
pinMode(BUTTON_PIN, INPUT_PULLUP);
if (digitalRead(BUTTON_PIN) == LOW) {
// Button is pressed
}
In this code snippet, the pinMode function configures the digital pin as an input with an internal pull-up resistor. The digitalRead function is then used to check if the button is pressed, indicated by the state being LOW.
Here’s a summary of the capabilities and functions of digital pins:
- Can be used for both input and output functions
- Read and write binary values (HIGH or LOW)
- Control external devices like LEDs or motors
- Read data from sensors or buttons
- Interface with other digital devices
Label | GPIO | Safe to use? | Reason |
D0 | 0 | No | must be HIGH during boot and LOW for programming |
TX0 | 1 | No | Tx pin, used for flashing and debugging |
D2 | 2 | No | must be LOW during boot and also connected to the on-board LED |
RX0 | 3 | No | Rx pin, used for flashing and debugging |
D4 | 4 | Yes | |
D5 | 5 | No | must be HIGH during boot |
D6 | 6 | No | Connected to Flash memory |
D7 | 7 | No | Connected to Flash memory |
D8 | 8 | No | Connected to Flash memory |
D9 | 9 | No | Connected to Flash memory |
D10 | 10 | No | Connected to Flash memory |
D11 | 11 | No | Connected to Flash memory |
D12 | 12 | No | must be LOW during boot |
D13 | 13 | Yes | |
D14 | 14 | Yes | |
D15 | 15 | No | must be HIGH during boot, prevents startup log if pulled LOW |
RX2 | 16 | Yes | |
TX2 | 17 | Yes | |
D18 | 18 | Yes | |
D19 | 19 | Yes | |
D21 | 21 | Yes | |
D22 | 22 | Yes | |
D23 | 23 | Yes | |
D25 | 25 | Yes | |
D26 | 26 | Yes | |
D27 | 27 | Yes | |
D32 | 32 | Yes | |
D33 | 33 | Yes | |
D34 | 34 | No | Input only GPIO, cannot be configured as output |
D35 | 35 | No | Input only GPIO, cannot be configured as output |
VP | 36 | No | Input only GPIO, cannot be configured as output |
VN | 39 | No | Input only GPIO, cannot be configured as output |
Analog Pins
The ESP32 supports 18 analog pins. However, only 15 are available in the DEVKIT V1 DOIT board (version with 30 GPIOs). The ESP32 offers a convenient set of analog pins that can be used to measure voltages ranging from 0 to 3.3 volts. These pins, labeled A0 to A19, provide the flexibility to connect analog sensors and devices, enabling you to gather and process real-world signals.
When it comes to utilizing the analog pins of the ESP32, it’s crucial to have a clear understanding of their purpose and how to use them effectively. Let’s take a closer look at some key aspects:
Analog Pin Functions
The primary function of the analog pins is to read analog signals. This means they can measure continuous voltage levels rather than digital signals, which are discrete and can only have two states (on or off).
By connecting various sensors, such as light sensors, temperature sensors, or potentiometers, to the analog pins, you can read their corresponding analog values. These values can then be used in your project to control outputs, make decisions, or provide feedback.
To better understand the capabilities of the analog pins, let’s take a look at the following table, which provides an overview of the ESP32 analog pins and their corresponding features:
Arduino Pin | ADC Channel | GPIO | Usable? |
---|---|---|---|
A0 | ADC1_CH0 | 36 | YES |
A3 | ADC1_CH3 | 39 | YES |
A4 | ADC1_CH4 | 32 | YES |
A5 | ADC1_CH5 | 33 | YES |
A6 | ADC1_CH6 | 34 | YES |
A7 | ADC1_CH7 | 35 | YES |
A10 | ADC2_CH0 | 4 | YES |
A11 | ADC2_CH1 | 0 | NO |
A12 | ADC2_CH2 | 2 | NO (LED Connected) |
A13 | ADC2_CH3 | 15 | YES |
A14 | ADC2_CH4 | 13 | YES |
A15 | ADC2_CH5 | 12 | NO |
A16 | ADC2_CH6 | 14 | YES |
A17 | ADC2_CH7 | 27 | YES |
A18 | ADC2_CH8 | 25 | YES |
A19 | ADC2_CH9 | 26 | YES |
Using Analog Pins
To utilize the analog pins of the ESP32, you need to follow a few key steps:
- Start by connecting your analog sensor or device to the desired analog pin. Ensure proper wiring and make sure the voltage levels are within the specified range of the analog pin.
- Next, configure the analog pin as an input using the appropriate code in your programming environment. This will allow the ESP32 to read the analog values.
- Once the pin is configured, you can use the built-in functions or libraries to read the analog values from the pin. These values can then be processed and used in your project.
By following these steps and understanding the capabilities of the analog pins, you can effectively capture and process analog data in your ESP32 projects.
Communication Pins
In this section, we will explore the communication pins of the ESP32 and how they enable seamless interaction with other devices. The ESP32 provides support for various communication protocols, including UART, SPI, and I2C, offering flexibility for a wide range of applications.
UART (Universal Asynchronous Receiver-Transmitter)
The UART protocol allows for serial communication between the ESP32 and other devices. It is especially useful for transmitting and receiving data in real-time. The ESP32 has multiple UART interfaces, each with its own set of transmit (TX) and receive (RX) pins.
SPI (Serial Peripheral Interface)
The SPI protocol facilitates high-speed, full-duplex communication between the ESP32 and external devices, such as sensors, displays, and SD cards. The ESP32 features dedicated pins for SPI communication, including a clock signal (SCK), a data input (MISO), a data output (MOSI), and a chip select (CS) pin.
I2C (Inter-Integrated Circuit)
The I2C protocol is a popular choice for low-speed communication between the ESP32 and various peripherals, such as sensors, EEPROMs, and LED drivers. With the ESP32, you can utilize the dedicated I2C pins, namely the Serial Data Line (SDA) and the Serial Clock Line (SCL), to establish reliable and efficient communication.
To provide you with a comprehensive visual reference, below is a detailed table that presents the communication pins of the ESP32:
Protocol | Pin Name | Description |
---|---|---|
UART | UART1 TX | Transmit pin for UART1 |
UART1 RX | Receive pin for UART1 | |
UART2 TX | Transmit pin for UART2 | |
UART2 RX | Receive pin for UART2 | |
SPI | SPI CLK | Clock signal pin for SPI |
SPI MOSI | Data output pin for SPI | |
SPI MISO | Data input pin for SPI | |
SPI CS | Chip select pin for SPI | |
I2C | I2C SDA | Serial Data Line for I2C |
I2C SCL | Serial Clock Line for I2C |
By consulting this table, you can easily identify the specific pins associated with each communication protocol on the ESP32, enabling you to interface effectively with external devices and unleash the full potential of your projects.
Power Pins
There are three power pins on the ESP32 board:
- Vin (Voltage IN): This pin is used to connect an external power supply to the ESP32 board. The voltage supplied through this pin should be within the recommended range (3.3V to 5V) to ensure proper functioning of the board.
- 3V3: This pin provides a regulated 3.3V output. It can be used as a power supply for external components or peripherals that require a 3.3V power source.
- GND (Ground): This pin is used to establish a common ground reference for the ESP32 board and the external devices or components connected to it. It ensures stability and proper functioning of the board.
Conclusion
In conclusion, this comprehensive guide has provided an in-depth understanding of the ESP32 pinout. We explored the digital pins, analog pins, communication pins, and power pins, and discussed their functions and capabilities. By familiarizing yourself with each pin, you can effectively utilize the ESP32’s full potential for your hardware projects.
Whether you’re a beginner or an experienced developer, a solid grasp of the ESP32 pinout is essential. By understanding how the pins operate and interact, you can confidently interface with external devices, capture analog data accurately, establish communication protocols, and supply the necessary power to your ESP32.
As you continue your hardware development journey, don’t underestimate the importance of the ESP32 pinout. It serves as a crucial foundation that enables you to create innovative and functional projects. So, take the time to study the various pins, experiment with different configurations, and unlock the endless possibilities that the ESP32 offers.
Write more, thats all I have to say. Literally, it seems as though you relied on the video to make your point. You definitely know what youre talking about, why waste your intelligence on just posting videos to your blog when you could be giving us something informative to read?
Your comment is awaiting moderation.